What is Javascript and What Does It Do? Javascript Examples
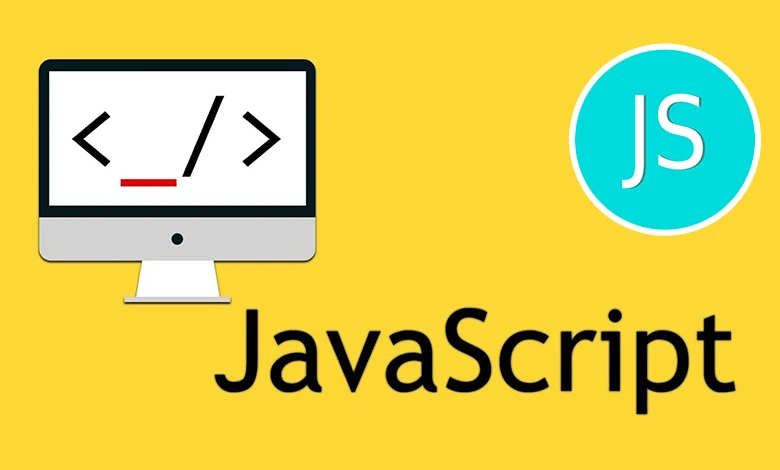
What is Javascript and What Does It Do? Javascript Examples. JavaScript is a synchronous and single-threaded programming (scripting) language that web developers use to create more dynamic interactions when developing web pages, applications, servers and even games. That is, it runs line by line and does not move to the next line until one line is executed and the process is complete.
What is Javascript?
JavaScript is a programming language that runs in web browsers. It was first developed by Netscape Communications in 1995. It was originally designed to be used only as client-side scripts on web pages, but it has been developed over time and has gained a wider usage area.
JavaScript is one of the client-side programming languages because it is run in the browser. Together with HTML and CSS, it enables web pages to be dynamic and interactive. It can change the content of pages, track user interactions and communicate with the server.
Outside of web development, JavaScript is now used on many different platforms. Thanks to platforms such as Node.js, it can also be used in server-side development. It is also widely used in areas such as mobile application development, desktop application development and game development.
JavaScript is an object-oriented programming language and is a dynamically typed language. This means that the types of variables are determined at runtime. Its syntax is influenced by languages such as C and Java, but it also has some differences.
What Does Javascript Do?
JavaScript is a fundamental component in the web development process and is used on almost every platform with web technologies today. It has a set of built-in code libraries, called JavaScript frameworks, specifically for developing web and mobile applications. Developers can use many open source or free frameworks to create platform-independent applications that can be built once and run on any device. Also, JavaScript code is lightweight and requires less processing, so developers can easily use it to build mobile apps.
Making Web Pages Interactive: JavaScript, along with HTML and CSS, makes web pages interactive and dynamic. It can detect events such as users clicking buttons, filling out forms, interacting with the mouse, and change the content on the page in response to these events. For example, it can perform functions such as changing text when a button is clicked, checking the correctness of a form, creating an animation or visualizing data.
Data Validation and Processing: JavaScript has the ability to validate and process the data that users enter into web forms. It can perform operations such as validating the data entered by users, formatting it, or checking whether it is a valid value. For example, it can check the validity of an e-mail address, verify that all fields of a form are filled in, or collect numeric values received from the user.
Communication with Web Services: JavaScript can be used to access web services (APIs) and exchange data. It can perform operations such as accessing data stored in a database or other web server or retrieving data from external sources. In this way, applications that work with live data can be created and dynamic content can be presented.
Animation and Graphical Effects: JavaScript can be used to create graphical effects and animations in the browser. For example, it can make an element scroll slowly, an image grow, or a visual effect appear when a button is clicked. In this way, impressive and visually rich web pages can be designed that enhance the user experience.
Game Development: JavaScript can be used to develop browser-based games. Together with technologies such as WebGL, it can create 3D graphics and implement game mechanics. It can integrate features such as user interaction, physics simulations, artificial intelligence into games.
Mobile Application Development: JavaScript can be used with various mobile application development frameworks to develop applications on mobile platforms such as iOS and Android. In this way, applications that run on different platforms can be created using the same code base.
Server-side Development: JavaScript can also be used on the server side with platforms such as Node.js. Server-side JavaScript can be used to perform server-side operations such as database operations, file operations, network requests. In this way, full-stack web applications can be created and it is possible to manage both the client side and the server side using a single language.
Javascript Examples
JavaScript is a code snippet used to develop web and mobile applications as a core component in the web development process.
We can list some examples that you can use in JavaScript as follows
Using Variables:
In JavaScript, variables are used to store values and perform operations. Below is an example of how a variable is defined and used:
// Variable declaration and assignment
var number = 5;
var name = “Ahmet”;
// Variable usage
console.log(counti); // 5
console.log(name); // “Ahmet”
// Update variable value
numberi = 10;
console.log(sayi); // 10
Condition Statements:
Condition statements allow different operations to be performed depending on a given condition. Below is an example of a simple if-else statement:
var numberi = 5;
if (numberi > 0) {
console.log(“Number is positive.”);
} else if (number < 0) {
console.log(“The number is negative.”);
} else {
console.log(“Number zero.”);
}
Loops:
In JavaScript, loops are used to run a specific block of code repeatedly. Here is an example of a for loop:
for (var i = 1; i <= 5; i++) {
console.log(i);
}
This loop will print the numbers from 1 to 5 on the screen.
Functions:
Functions are used to perform a specific function. Below is an example of a function:
function sum(a, b) {
var result = a + b;
return result;
}
var total = sum(3, 4);
console.log(sum); // 7
In this example, we define a function called sum and take two parameters and return the sum of these parameters. Then we call the function and assign the result to a variable called sum.
Series:
Arrays are used to hold multiple values of the same type. Below is an example of an array:
var colors = [“red”, “blue”, “green”];
console.log(colors[0]); // “red”
console.log(colors[1]); // “blue”
console.log(colors[2]); // “green”
In this example, we define an array called colors and then access its elements using an index.
Since JavaScript is a very broad language with more complex features and use cases, you should not be limited to these examples. Studying and practicing the official JavaScript documentation will help you learn more details of the language.
You may be interested 👇
👉 Top 5 Sites to Learn Web Programming 2023
👉 What is Localhost and What Does It Do?
👉 What is an IP Address and What Does It Do? IP
👉 What is Domain Authority (DA), Page Authority (PA)?
👉 Click to follow the Student Agenda on Instagram